How to use Python in a Bash script
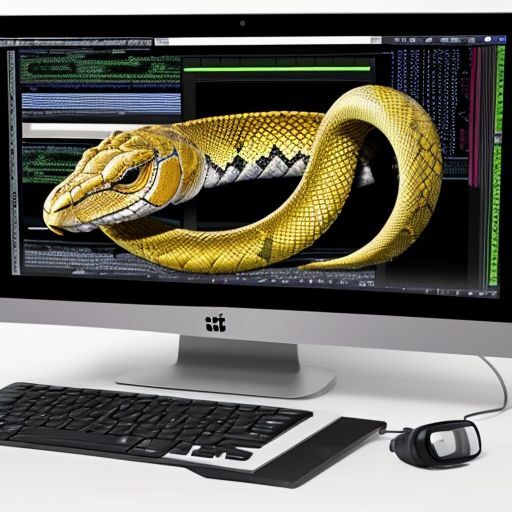
Bash is a versatile language, but sometimes I would rather do string processing in Python. Now I could make a custom Python script and call it from bash, but doing things like that will quickly clutter up your filesystem. If you embed the Python script in your Bash script, you won't have an extra file.
To embed Python, the general technique is to use the "-c" option and start the Python command with an "if" statement in order to set the indentation level. Use single quotes around the python script to suppress bash variable expansion.
function getfilename {
# if filepath is /foo/bar.baz
#usage: $(getfilename filepath) --> bar.baz
python3 -c 'if 1: #<--for the indentation level
import sys
import os
arg=sys.argv[1]
ss=os.path.basename(arg)
print(ss)
' "$1" ;
}
You can also use a "here document":
python3 <<-'EOF'
print("An Example of how to run python in bash.")
EOF
The single quotes around the EOF suppress Bash variable expansion. The dash: "-" allows you to use tabs for indenting your "here document".
You can take this technique even further by embedding Python in Bash in a Makefile!
So, yeah, I did this and it changed my life.